Integration
Detailed Integration Workflow Diagram
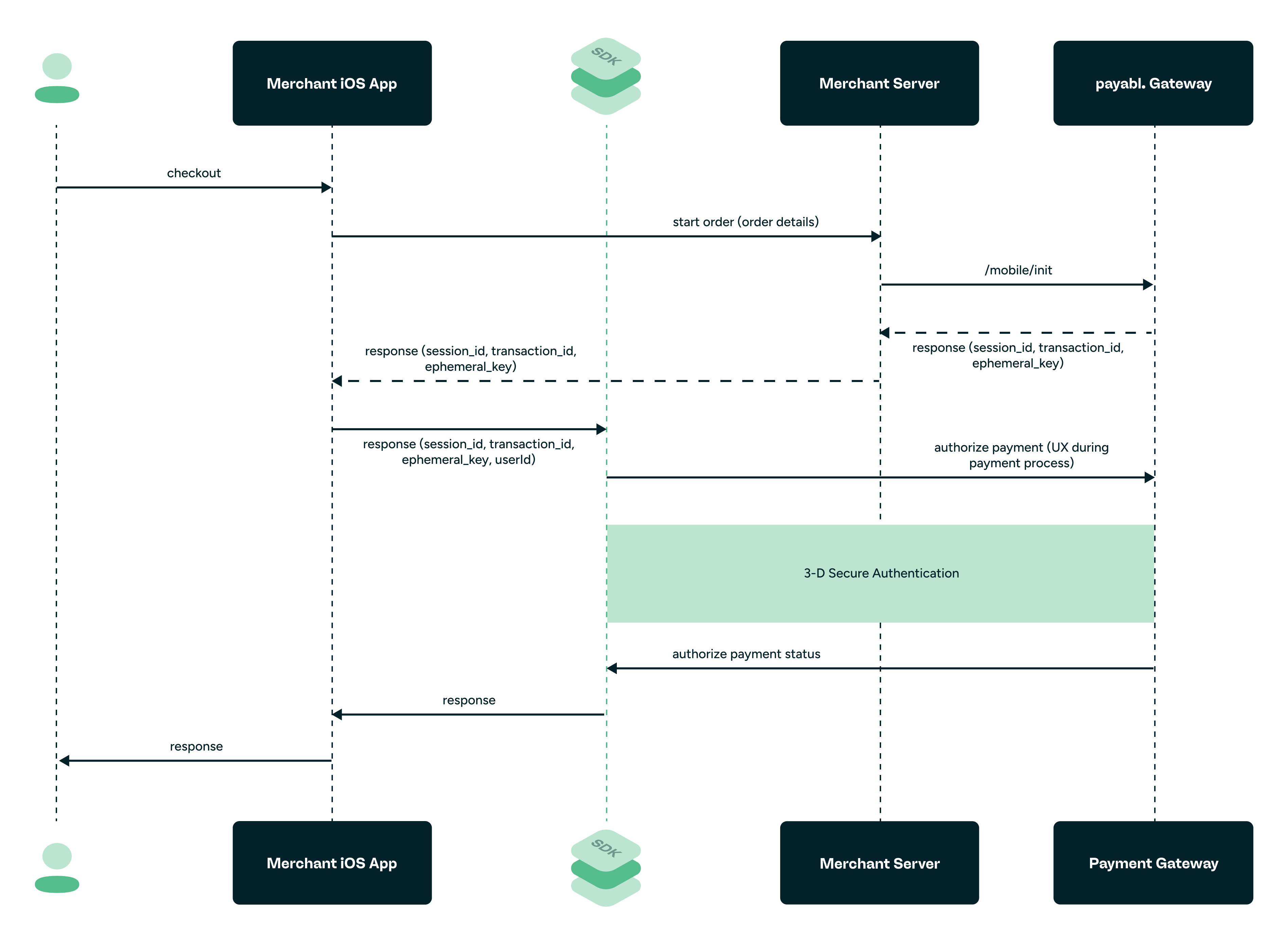
iOS SDK - Integration Workflow (Card Payment)
Using the SDK
The Payabl SDK is designed to handle the entire payment flow, including UI, card input, 3D Secure authentication, and transaction confirmation. Your app is only responsible for triggering the flow using credentials provided by your backend.
Get the Session ID First
This example assumes your backend has already created a session and securely returned the necessary credentials to the app.
1. Import the SDK
import PayablMerchantSDK
2. Integrate iOS SDK Payment Page
-
SwiftUI Project:
class DemoCartViewModel: ObservableObject { @Published var paymentPage: PBLPaymentPage? let backendCheckoutUrl = = URL(string: "backend_endpoint/payment_page")! func preparePaymentPage() { var request = URLRequest(url: backendCheckoutUrl) request.httpMethod = "POST" let task = URLSession.shared.dataTask(with: request, completionHandler: { [weak self] (data, response, error) in guard let data = data, let json = try? JSONSerialization.jsonObject(with: data, options: []) as? [String : Any] else { return } let sessionId = json["sessionId"] let transactionId = json["transactionId"] let ephemeralKey = json["empheralKey"] let merchantId = json["merchantId"] let config = PBLConfiguration( sessionId: sessionId, transactionId: transactionId, ephemeralKey: ephemeralKey, merchantId: merchantId, customerId: currentUser.shared.email, // any customer identifer can work environment: .sandbox ) self.paymentPage = PBLPaymentPage(configuration: config) } } func didFinishCheckout(result: PBLPaymentResult) { switch result { case .canceled: print("Client has canceled the checkout proccess") case .completed: print("Client has successfuly completed checkout") case .failed(let error): print("Failed due to: ", error.localizedDescription) default: fatalError() } } } struct ContentView: View { let demoCartViewModel = DemoCartViewModel() var body: some View { VStack { PBLPaymentButton(paymentPage: demo.paymentPage) { result in demoCartViewModel.didFinishCheckout(result: result) } content: { Text("Checkout") .padding() } } .foregroundStyle(.white) .background(.black) .clipShape(RoundedRectangle(cornerRadius: 10)) .padding() .onAppear { demoCartViewModel.preparePaymentPage() } } }
-
UIKit Project:
class ViewController: UIViewController { let pblPaymentPage: PBLPaymentPage? override func viewDidLoad() { super.viewDidLoad() let checkoutButton = UIButton(type: .system) checkoutButton.setTitle("Checkout", for: .normal) checkoutButton.setTitleColor(.white, for: .normal) checkoutButton.backgroundColor = .blue checkoutButton.layer.cornerRadius = 8 checkoutButton.titleLabel?.font = UIFont.boldSystemFont(ofSize: 18) checkoutButton.frame = CGRect(x: 50, y: 200, width: 200, height: 50) self.view.addSubview(checkoutButton) checkoutButton.addTarget(self, action: #selector(payableOrder), for: .touchUpInside) } @objc func payableOrder() { var request = URLRequest(url: backendCheckoutUrl) request.httpMethod = "POST" let task = URLSession.shared.dataTask(with: request, completionHandler: { [weak self] (data, response, error) in guard let data = data, let json = try? JSONSerialization.jsonObject(with: data, options: []) as? [String : Any] else { return } let sessionId = json["sessionId"] let transactionId = json["transactionId"] let ephemeralKey = json["empheralKey"] let merchantId = json["merchantId"] let config = PBLConfiguration( sessionId: sessionId, transactionId: transactionId, ephemeralKey: ephemeralKey, merchantId: merchantId, customerId: currentUser.shared.email, // any customer identifer can work environment: .sandbox ) pblPaymentPage = PBLPaymentPage(configuration: config) pblPaymentPage.present(from: self) { status in switch status { case .canceled: print("Client has canceled the checkout proccess") case .completed: print("Client has successfuly completed checkout") case .failed(let error): print("Failed due to: ", error.localizedDescription) default: fatalError() } } } }
No additional configuration or setup is required. The SDK handles the full flow.
Testing the Integration
You can test the SDK integration using your sandbox credentials. Make sure your backend calls the sandbox endpoints provided by the Payabl gateway.
- Use the sandbox environment
https://pay4.sandbox.payabl.com/pay/mobile/init
for testing your implementation. - Ensure you verify all payment scenarios:
- Successful checkout.
- Cancelled checkout.
- Failed checkout.
Updated about 2 months ago