Integration Steps
Start with steps when preliminary steps (Get Session ID) in the Initiating Session are successfully done.
Detailed Workflow with Description
Here is a detailed workflow as well as a step-by-step description of how the integration with the Web SDK works:
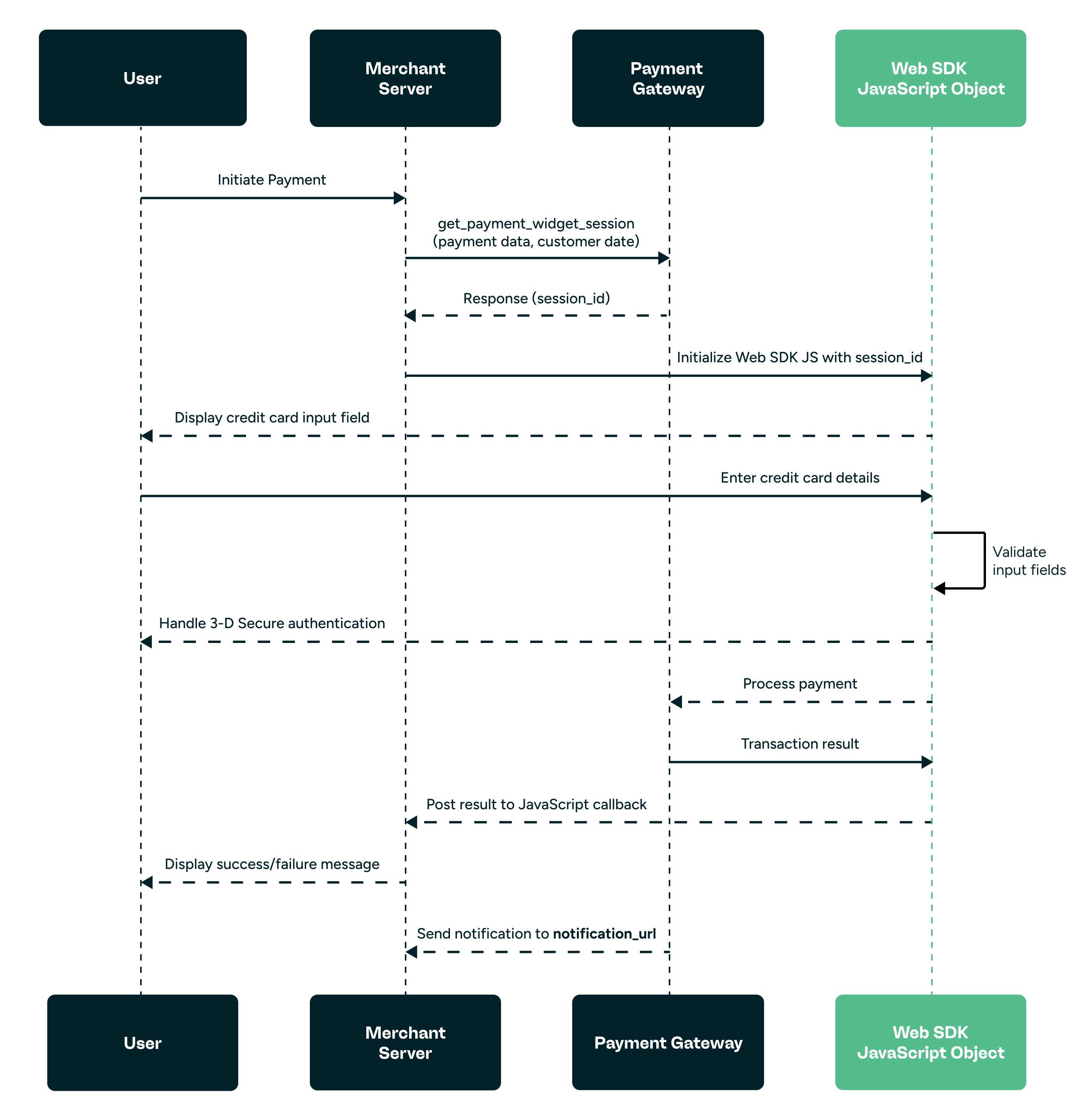
Web SDK - Detailed Workflow
Detailed Workflow Description
- In the server to server
get_payment_widget_session
payment request, the payment gateway should receive all required and optional parameters (Payment Data and Customer Data) from the merchant’s server for payment processing. - The payment gateway sends the merchant response that includes the initial URL (
session_id
parameter) for using with the Web SDK JavaScript. This is only possible if merchant's server receives a successful response from the payment gateway after theget_payment_widget_session
payment request. - Once it has been successfully created in the Web SDK, the customer will be presented with the credit card input fields.
- After all the fields are completely and correctly filled in, the customer can proceed and submit the payment.
- If the card is required for 3-D Secure authentication, the Web SDK handles it seamlessly. No need for the merchant to take any additional action.
- Once the transaction is complete, the Web SDK will post the result of the transaction to a JavaScript callback provided by the merchant.
- The merchant uses this message to inform the customer of a success or failure and to take the necessary actions to take control of the page.
- The merchant should not use the result of the callback notification to update its system. Instead, there will be a notification sent to the
notification_url
provided in the server-to-server request.
Web SDK Display Modes
Our Web SDK offers two display modes for integrating the payment widget into your website: Modal and Inline. The payment widget mode is configured in payabl.object
and is set by the widgetMode
parameter. The default value is "inline."
Modal Mode
In modal mode, the payment form appears as pop-up overlay on top of your webpage. This mode is useful when you want to keep the main content of your webpage visible while the user completes the payment process. The modal includes a credit card form and a submit button, which can be customized with text like "Pay $1.23"
Inline Mode
In inline mode, the payment form is embedded directly within your webpage. This mode seamlessly integrates the credit card form into your page layout, making it part of the overall design. Users can fill out the payment details without leaving the current page view.
Both modes handle the full payment process, including 3-D Secure authentication if required, and provide callbacks to post the transaction result back to your website. You set one of these modes for your website while initiating payabl API. Please refer to Step 4 of the following integration step-by-step guide.
Steps to Integrate with the Web SDK
Step 1: Get a Unique Web SDK sessionid
sessionid
Put the Signature to server-to-server request to get the sessionid
. Refer to Payment Data and Customer Data Parameters to get sessionid
POST https://sandbox.payabl.com/pay/payment/get_payment_widget_session?merchantid=gateway_test&amount=1.23¤cy=EUR&[email protected]&customerip=127.1.1.1&shop_url=https://127.0.0.1:5500&signature=7c57c5629154d8d39c966cb1e5c4068826c18523
Step 2: Include the SDK Script
Reference the Web SDK library in your HTML form:
...
<script type="text/javascript" src="https://pay4.sandbox.payabl.com/hpp/js/sdk/latest/payabl_sdk.js"></script>
...
Step 3: Add HTML Placeholder in Your HTML Form
...
<div id="creditcard-placeholder">
<!-- payabl. widget will be inserted here. -->
</div>
...
Step 4: Initiate payabl. API
Create an instance of the payabl. API in your JavaScript code using a payabl. object.
Important
To avoid CORS errors, ensure that the Web SDK widget is hosted on the same page as the
shop_url
, and theshop_url
value passed during the Web SDK initialization.
payabl.initialize({
sessionId: '538b891512c5aa708899d4d34d07ea5f1890e2d8',
env: 'sandbox',
widgetMode: "modal",
modalPayButtonText: "Pay $1.23",
callback : callbackNotification,
});
Switching from Sandbox to Live Environment
When you are ready to go live, change the env
parameter to live
in your JavaScript initialization code:
Payabl.initialize({
sessionId: 'YOUR_SESSION_ID', // REQUIRED. Received during server-side authentication
env: 'live', // REQUIRED. Set this to 'live' for the production environment
// other parameters...
});
Additionally, update the Web SDK URL to the production version:
<script src="https://pay4.payabl.com/hpp/js/sdk/latest/payabl_sdk.js"></script>
Note
Please remember, in the sandbox environment, the
shop_url
parameter must always be set tohttps://127.0.0.1:5500
value. No test transactions can be performed in the production environment.
Here are properties of payabl. initialize
and their descriptions:
Property | Values | Default Value | Description |
---|---|---|---|
sessionId | N/A | ID generated during server-to-server init API request. See init section for details. | |
env | sandbox | live | live | sandbox – this is used during the integration phase. live – used to send live cards numbers. |
widgetMode | inline | modal | inline | This controls the display mode of the credit card. The “inline” mode seamlessly embeds the credit card form into the page. The “modal” mode shows a modal form with credit card form and submit button. |
modalPayButtonText | “Pay” | This is used together with “modal” mode to customize the text of the button. See screenshots section for an example. | |
callback | JavaScript function | N/A | After the payment is completed this is used to post the result back to the merchant website JavaScript. See callback section for details. |
Step 5 (optional): Design Customization
Set Web SDK style in your JavaScript code. For the detailed description of how to change Web SDK style view, please refer to Design Customization section.
payabl.setStyle()
const customStyle = {
input: {
color: "green",
fontSize: "12px",
borderRadius: "0px",
borderColor: "red",
backgroundColor: "pink",
height: "70px",
padding: "5px",
pseudoStyles: {
hover: {
color: "red",
backgroundColor: "lightgray"
}
},
},
select: {
color: "green",
fontSize: "12px",
borderRadius: "0px",
borderColor: "red",
backgroundColor: "pink",
height: "70px",
padding: "5px",
},
label: {
color: "purple",
fontSize: "12px",
marginTop: "0px",
marginBottom: "0px",
},
tabSlider: {
height: "60px",
backgroundColor: "cyan",
borderColor: "red",
borderWidth: "1px",
borderStyle: "solid",
borderRadius: "0px",
},
savedCardBox: {
borderColor: "cyan",
borderWidth: "1px",
borderStyle: "solid",
borderRadius: "0px",
backgroundColor: "yellow",
},
slider: {
borderColor: "cyan",
borderWidth: "1px",
borderStyle: "solid",
borderRadius: "0px",
backgroundColor: "yellow",
},
savedCardsBox: {
minWidth: "300px",
width: "420px",
marginTop: "160px",
},
newCardBox: {
minWidth: "300px",
width: "420px",
marginTop: "160px",
},
};
Step 6: Render the Widget
This will embed the credit card form in the browser
payabl.create()
payabl.create(document.getElementById('creditcard-placeholder'));
Step 7: Final Step: Submit the Payment
Attention
In case of using “modal” mode it renders the payment form in the user's browser, and you must not do the final step (Step 7).
Submit the Payment by calling createPayment
method. Add an event listener to a button. If you use “inline” mode, this step is required.
payabl.createPayment
let pay_button = document.getElementById("submitButton");
pay_button.addEventListener("click", payabl.createPayment, false);
To check the response parameters and their formats please refer to Response Parameters description
Updated 13 days ago